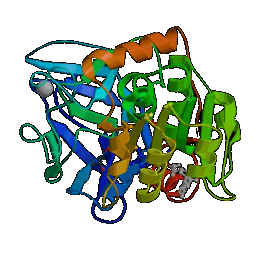
Generative Creativity - Lecture 2:
The basics
What it's about
There is ongoing debate about what creativity really is, or at
least what the concept picks out.
But this course side-steps that issue, taking creativity to be any
process which produces an especially valued artefact.
The key distinction will be between `hands-on' and `hands-off' versions
of the process.
- Hands-on creativity: production of a valued artefact by direct
action.
- Hands-off creativity: production of a valued artefact by indirect
action, i.e., manipulation of a generative mechanism.
The course focusses on the latter approach with attention focussing
particularly on the possibilities opened up by modern, computational
methods.
Basic questions
To make progress with any application of generative creativity, we need
detailed answers to specific questions.
- How does the generative process work?
- How are its products valued?
- Why are they valued?
So concerning production of images, say, we would want to know
- what is the process by which a particular type of image is
constructed?
- how might it be valued?
- are there different types of valuation?
- can we maximise value somehow?
Application areas
Generative approaches are increasingly common in these areas.
- Music (the biggest area at present)
- Images
- Video and animation
- Stories
- Jokes
- Theories, analogies and models
- Poetry
- Interactive experiences combining several of the above
All approaches involve use of models.
Some attempt to build a model from examples.
Basic equipment
Java applets provide an excellent, zero-cost, genre-free framework for
presenting generative media over the web.
But they are quite complex to use and some of the `fine details' of
object-oriented programming are not really required.
We need some specific methods to make life easy.
Hello World applet - Java code
import javax.swing.*;
import java.awt.*;
class AppletPanel extends JPanel {
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawString("Hello World", 10, 20);
}
}
public class Hello extends JApplet {
public void init() {
getContentPane().add(new AppletPanel());
}
}
Hello World applet - HTML code
Make a file using a text editor called `Hello.html' containing
<html>
<body>
<applet code="Hello.class" width=128 height=128>
</body>
</html>
You can do this for any applet. The crucial details is the value of
the 'code' parameter. This must be the name of the relevant class
file.
Hello World applet in action
To run the applet, first make sure you have html file and the class file
in the same folder. Then simply open `Hello.html' in a web browser.
Alternatively, you can run the applet as a standalone application using
the `appletviewer' command from the command prompt:
appletviewer Hello.html
Fonts and backgrounds
To change the background color, insert something like
setBackground(Color.blue);
inside the paintComponent definition.
To change the pen color insert something like
g.setColor(Color.yellow);
before the drawString call.
Mouse and keyboard interaction
If we want the applet to be interactive, we need to add MouseListener
functionality.
This involves defining methods which `listen' out for certain types of
button-press.
For full details, look at the documentation for `MouseListener'.
(This is most easily done by googling `MouseListener', or `Java 5.0
MouseListener' if you want specific documentation for version 5,
say.) Look at the documentation for `KeyListener' to see how to
make an applet listen to the keyboard.
Interactive Hello World applet
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
class HelloWorldPanel extends JPanel implements MouseListener {
MouseEvent event;
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.drawString("Hello World", 10, 20);
if (event != null) g.fillOval(event.getX(), event.getY(), 20, 20);
}
public void mousePressed(MouseEvent event) {
this.event = event;
repaint();
}
public void mouseReleased(MouseEvent event) { }
public void mouseClicked(MouseEvent event) { }
public void mouseEntered(MouseEvent event) { }
public void mouseExited(MouseEvent event) { }
}
public class HelloWorld extends JApplet {
public void init() {
HelloWorldPanel appletPanel = new HelloWorldPanel();
appletPanel.addMouseListener(appletPanel);
appletPanel.setBackground(Color.pink);
getContentPane().add(appletPanel);
}
}
Interactive applet
Summary
- For this course, creativity is taken to be any process which
produces an especially valued artefact. No particular emphasis on
`novelty' or `appropriateness' since these are implicit in `especially
valued'.
- Key questions for the course are `how does the mechanism work?', `how
are the products valued?' and `why?'
- Using Java applets for maximum power, flexibility and accessibility.
But using shortcuts where possible.
Exercises
- Look at the documentation for the Color class. Then modify the applet
so that it uses one of the other colors predefined by this class.
- Modify the applet so that clicking in the bottom, right corner causes
the Java `beep' method to be called: Toolkit.getDefaultToolkit().beep();
- Add a MouseMotionListener to the applet and modify the code so that
you can drag the blob of color around with the mouse.
Resources
- Sun applet tutorial:
http://java.sun.com/docs/books/tutorial/uiswing/components/applet.html
- Relevant Java documentation: `JApplet' `MouseListener',
`KeyListener', `JPanel', `Graphics'. You can pick the documentation
pages up using google. For an unsual word like `MouseListener',
just specifying this as the search string should be enough to pull
up the class documentation (as first result), provided you use the
right mix of case. But for more common words (like `Graphics') you
need to narrow down the search by inluding something like `Java
5.0' at the front.
Page created on: Wed Jan 13 12:09:42 GMT 2010
Feedback to Chris Thornton